In order to allow users to log in to the system, you will likely need an HTML form on your page to accept the user's handle and password. The form helper (Solar_View_Helper_Form) available to Solar_View makes light work of adding the form to any view or layout.
![]() |
Note |
---|---|
Please review the chapter on Views and Layouts for more information on working with views and layouts. |
The logic for the authentication form goes something like this:
Figure 7.1. Authentication logic when using an HTML form
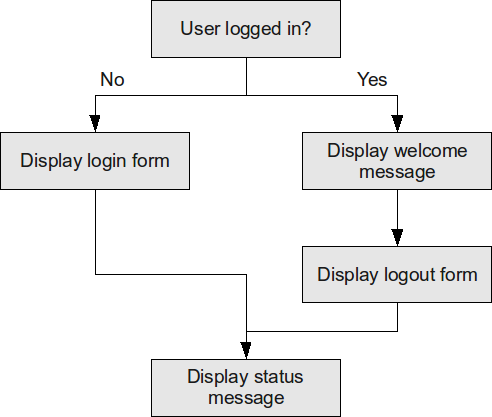
If the user is not logged in, the login form is displayed. If the user is logged in, you may see a welcome message, and then a logout form with a "Sign Out" button. Below the login or logout form may be a status message, such as "Welcome back!" or "Your username and password did not match. Please try again.".
The code to represent this logic in your view might look like the
following. (Assume that the public property $user
is a Solar_User
object available to the view.)
<div id="user">
<?php
if ($this->user->auth->isValid()) {
// the user is logged in, show a logout form
echo "<div>\n"
. $this->getText('TEXT_AUTH_USERNAME') . "\n"
. $this->escape($this->user->auth->handle) . "\n"
. "</div>\n";
echo $this->form()
->addProcess('logout')
->decorateAsDivs()
->fetch();
} else {
// the user is not logged in, show a login form
echo $this->form()
->text(array(
'name' => 'handle',
'label' => 'LABEL_HANDLE',
))
->password(array(
'name' => 'passwd',
'label' => 'LABEL_PASSWD',
))
->addProcess('login')
->decorateAsDivs()
->fetch();
}
// always show the authentication status
$status = $this->user->auth->getStatusText();
if ($status) {
echo '<div class="status">'
. $this->escape($status)
. "</div>\n";
}
?>
</div>
So, if the user is logged in
(as noted by the result of the $this->user->auth->isValid()
call), then a "Signed in
as ..." message will appear with a "Sign Out" button below.
Additionally, any status messages such as "Welcome back!" will appear
below the logout button. (These status messages are automatically
generated by the Solar_Auth
adapter based on the success or failure of its automated login and
logout processing.)
If, however, the user is not logged in, or the authentication attempt failed, then a login form will appear with any status messages below. See the images below as examples.
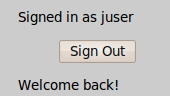
The image above shows a successful login. Note the welcome message above the form, and the status message below.
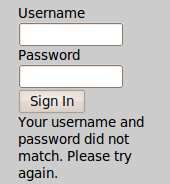
The image above shows a failed login. Note the status message below the form.